Javascript - DOM
HTML文件的階層結構在DOM中是以樹結構來表達。樹的節點是代表文件中各種型態的內容。
<html>
<head>
<title>Sample Document</title>
</head>
<body>
<h1>An HTML Document</h1>
<p>This is a <i>Simple</i> document.
</body>
</html>
以DOM的觀點來看這份文件,結構如下
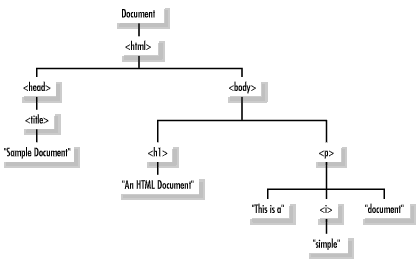
- Node物件的childNodes屬性會傳回該節點的子節點清單。
- firstChild, lastChild,nextSibling, previousSibling,parentNode屬性讓你尋訪樹結構中的節點的手段
- appendChild(), removeChild(), replaceChild(), insertBefore()可以在文件數中新增移除節點
介面 NodeType常數 nodeType之值
Element Node.ELEMENT_NODE 1
Text Node.TEXT_NODE 3
<head>
<script>
function counterTags(n){
var nTags = 0;
if(n.nodeType==1){
nTags++;
// alert(n.tagName);
}
var children = n.childNodes;
for(var i=0; i<=children.length-1; i++){
nTags += counterTags(children[i]);
}
return nTags;
}
</script>
</head>
<body onload = "alert('this document has '+counterTags(document)+' tags')">
<h1>This is a <i>sample</i> document.</h1>
</body>
會alert "this document has 7 tags",分別是HTML,HEAD,TITLE,SCRIPT,BODY,H1,I,
雖然沒有HTML, TITLE不過也會計算進去。
找出文件中特定的元素
getElementsByTagName("body")[0]
//getElementByTagName()傳回一個NodeList物件。視為陣列
如果想對文件中的第四段落做些什麼事
var myParagraph = document.getElementsByName("p")[3]
不是最佳做好->在文件的開頭新插入一段,程式就不能用了。最好是給一個id標籤,替元素指定獨一無二的名稱。
<p id="specialParagraph">
就可以用var myParagraph = document.getElementById("specialParagraph")
注意getElementById()不像getElementsByTagName()傳回含有元素的陣列。因為每個id特性之值都是唯一的,getElementsById()只會傳回一個吻合id特性的單一元素。
可以使用getElementById()來找出特定元素,再用getElementsByTagName()來找出該元素下特定型態的所有子孫節點。
//在文件中找出特定的表格元素,在計算其資料列數目
var tableOfContents = document.getElementById("TOC");
var rows = tableOfContents.getElementByTagName("tr");
var numrows = rows.length;
修改文件
利用document.createTextNode()來建立新的Text節點
<script>
// This function recursively looks at node n and its descendants,
// replacing all Text nodes with their uppercase equivalents.
function uppercase(n) {
if (n.nodeType == 3 /*Node.TEXT_NODE*/) {
// If the node is a Text node, create a new Text node that
// holds the uppercase version of the node's text, and use the
// replaceChild() method of the parent node to replace the
// original node with the new uppercase node.
var newNode = document.createTextNode(n.data.toUpperCase());
var parent = n.parentNode;
parent.replaceChild(newNode, n);
}
else {
// If the node was not a Text node, loop through its children,
// and recursively call this function on each child.
var kids = n.childNodes;
for(var i = 0; i < kids.length; i++) uppercase(kids[i]);
}
}
</script>
<!-- Here is some sample text. Note that the p tags have id attributes -->
<p id="p1">This <i>is</i> paragraph 1.</p>
<p id="p2">This <i>is</i> paragraph 2.</p>
<!-- Here is a button that invokes the uppercase() function defined above -->
<!-- Note the call to Document.getElementById() to find the desired node -->
<button onclick="uppercase(document.getElementById('p1'));">Click Me</button>
利用document.createElement建立新的元素(html tag)
<script>
// This function takes a node n, replaces it in the tree with an Element node
// that represents an html <b> tag, and then makes the original node the
// child of the new <b> element.
function embolden(node) {
var bold = document.createElement("b"); // Create a new <b> Element
var parent = node.parentNode; // Get the parent of node
parent.replaceChild(bold, node); // Replace node with the <b> tag
bold.appendChild(node); // Make node a child of the <b> tag
}
</SCRIPT>
<!-- A couple of sample paragraphs -->
<p id="p1">This <i>is</i> paragraph #1.</p>
<p id="p2">This <i>is</i> paragraph #2.</p>
<!-- A button that invokes the embolden() function on the first paragraph -->
<button onclick="embolden(document.getElementById('p1'));">Embolden</button>
<script>
function changelink(){
document.getElementById('myAnchor').innerHTML = "visit W3Schools";
document.getElementById('myAnchor').href = "http://www.w3schools.com";
document.getElementById('myAnchor').target = "_blank";
}
</script>
<a id="myanchor" href="http://www.microsoft.com">Visit Microsoft</a>
<input type="button" onclick=changelink() value="change link">
</pre>
沒有留言:
張貼留言